We always seem to meet people at conferences who are looking for a Bluetooth gateway solution. Golioth is the universal connector for IoT, and today we’re going to take a look at one way to extend that to your Bluetooth devices.
Luckily, Bluetooth is well supported by Zephyr. We’re going to run some sample code, then customize it to use Golioth as the cloud connection for a Bluetooth gateway device. Our target hardware is an nRF9160-DK as the gateway (we’ll refer to this as the central), and an nRF52840-DK as the sensor (which we’ll call the peripheral).
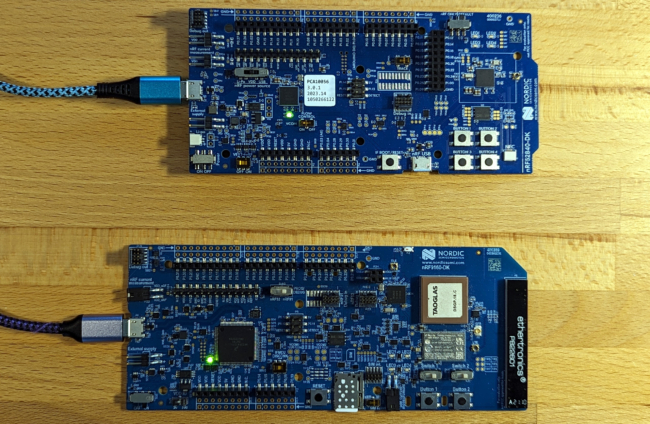
Upper: nRF52840-DK as a Bluetooth health temperature sensor
Lower: nRF9160-DK as a Bluetooth-to-Cellular gateway
We’re using the nRF Connect SDK version of Zephyr. You can follow our NCS with Golioth documentation to install it locally if you have not already done so.
HCI low power UART
We chose the nRF9160-DK because it has both an nRF9160 to provide a cellular connection, and an nRF52840 as a Bluetooth radio. The nRF9160 will run the show, communicating with the nRF52840 over UART. Nordic has an HCI low power UART sample that we will compile and run on the nRF52840 (the one that’s on the nRF9160-DK board) before we work on the central code for the nRF9160.
1. Build the HCI low power UART
Note that this build command compiles code for the nRF52840 chip that is on the nRF9160-DK development board.
cd ~/golioth-ncs-workspace/nrf/samples/bluetooth/hci_lpuart west build -b nrf9160dk_nrf52840 .
2. Move the Prog/Debug switch and flash
Locate the PROG/DEBUG
switch on the nRF9160-DK and move it to the nRF52
position. This controls a mux that points at the Bluetooth chip. Use west to flash the firmware:
➜ west flash -- west flash: rebuilding ninja: no work to do. -- west flash: using runner nrfjprog Using board 960088581 -- runners.nrfjprog: Flashing file: /home/mike/golioth-compile/golioth-firmware-sdk/nrf/samples/bluetooth/hci_lpuart/build/zephyr/zephyr.hex [ #################### ] 4.820s | Erase file - Done erasing [ #################### ] 1.207s | Program file - Done programming [ #################### ] 1.227s | Verify file - Done verifying Enabling pin reset. Applying pin reset.
When done, move the programming switch back to nRF9160 so it’s ready for the next step.
Load the central_ht sample on the nRF9160-DK
Zephyr includes a Health Temperature Service sample application which we’ll use as our hello world. Let’s compile and flash the “central” part of that sample.
1. Add board files to the central_ht sample code
Navigate to the central_ht sample code in the Zephry tree:
cd ~/golioth-ncs-workspace/zephyr/samples/bluetooth/central_ht/
We need to add a boards directory and create a conf file and and two overlay files for the nRF9160 in that directory. These configure the board to use the HCI low power UART firmware we flashed in the previous section as the Bluetooth radio (think of it as a secondary modem controlled by the application processor on the nRF9160).
In total we add 3 files:
- nrf9160dk_nrf9160_ns.conf
- nrf9160dk_nrf9160_ns.overlay
- nrf9160dk_nrf9160_ns_0_14_0.overlay
# HCI low power UART CONFIG_NRF_SW_LPUART=y CONFIG_NRF_SW_LPUART_INT_DRIVEN=y CONFIG_UART_2_ASYNC=y CONFIG_UART_2_INTERRUPT_DRIVEN=n CONFIG_UART_2_NRF_HW_ASYNC=y CONFIG_UART_2_NRF_HW_ASYNC_TIMER=2
#include <nrf9160dk_nrf52840_reset_on_if5.dtsi> / { chosen { zephyr,bt-uart=&lpuart; }; }; &gpiote { interrupts = <49 NRF_DEFAULT_IRQ_PRIORITY>; }; &uart2 { current-speed = <1000000>; status = "okay"; /delete-property/ hw-flow-control; pinctrl-0 = <&uart2_default_alt>; pinctrl-1 = <&uart2_sleep_alt>; pinctrl-names = "default", "sleep"; lpuart: nrf-sw-lpuart { compatible = "nordic,nrf-sw-lpuart"; status = "okay"; req-pin = <21>; /* <&interface_to_nrf52840 3 0>; */ rdy-pin = <19>; /* <&interface_to_nrf52840 2 0>; */ }; }; &pinctrl { uart2_default_alt: uart2_default_alt { group1 { psels = <NRF_PSEL(UART_TX, 0, 18)>, <NRF_PSEL(UART_RX, 0, 17)>; }; }; uart2_sleep_alt: uart2_sleep_alt { group1 { psels = <NRF_PSEL(UART_TX, 0, 18)>, <NRF_PSEL(UART_RX, 0, 17)>; low-power-enable; }; }; };
It’s important to add this second overlay file that properly maps the reset line for newer nRF9160-DK boards:
/* Use the reset line that is available starting from v0.14.0 of the DK. */ #include <nrf9160dk_nrf52840_reset_on_if9.dtsi>
2. Build and flash the central_ht sample code
west build -b nrf9160dk_nrf9160_ns . west flash
If you get an error when trying to flash the board, ensure you have the PROG/DEBUG
switch in the nRF91
position.
The nRF9160-DK will immediately begin scanning for compatible Bluetooth human temperature services. Let’s set up one of those next.
Load the peripheral_ht sample on the nRF52840-DK
The previous steps complete the “central” part of the Bluetooth equation which will scan for available sensors. Now we need to create a “peripheral”, which is the sensor that will advertise itself and serve temperature readings from a sensor.
Building and flashing this sample code is very straight-forward:
cd ~/golioth-ncs-workspace/zephyr/samples/bluetooth/peripheral_ht/ west build -b nrf52840dk_nrf52840 . west flash
Monitor the output
Connect to the nRF9160-DK over serial and you should see the device scan for a compatible peripheral, connect to it, and begin taking temperature readings:
*** Booting nRF Connect SDK v2.5.2 *** Temperature 23.75C. Connected: E9:E3:F1:6D:A9:87 (random) [ATTRIBUTE] handle 25 [ATTRIBUTE] handle 26 [ATTRIBUTE] handle 28 [SUBSCRIBED] Temperature 23.75C. Temperature 23.75C. Temperature 23.75C. Temperature 23.75C. Temperature 24C.
Next up, let’s connect Golioth and send these readings to the cloud!
Add Golioth to the project
To Golioth to this project we need three things:
- Ensure Golioth is installed as a Zephyr module
- Add Kconfig symbols to enable nRF9160 cellular and to add Golioth
- Add a few API calls to the central_ht code to connect to Golioth and push the temperature reading to the cloud
In this section we’ll be working with the Zephyr central_ht sample so let’s switch to that directory:
cd ~/golioth-ncs-workspace/zephyr/samples/bluetooth/central_ht/
1. Add Golioth as a Zephyr Module
If you followed our getting started guide for NCS, this is already done. If not, you can follow the Adding the Golioth Firmware SDK to an Existing Zephyr West Project section of our SDK readme.
2. Add Kconfig symbols for cellular and Golioth
For these changes, we’ll crib a lot of code from Golioth’s LightDB Stream sample since we’re using that service to stream the temperature to the cloud.
Add the contents of the nRF9160-DK board file from the Golioth lightdb_stream sample to the board file you previously created in the central_ht Zephyr sample. Here’s what that file should look like now:
# General config CONFIG_HEAP_MEM_POOL_SIZE=4096 CONFIG_NEWLIB_LIBC=y # Networking CONFIG_NET_SOCKETS_OFFLOAD=y CONFIG_NET_IPV6=y CONFIG_NET_IPV6_NBR_CACHE=n CONFIG_NET_IPV6_MLD=n # Increase native TLS socket implementation, so that it is chosen instead of # offloaded nRF91 sockets CONFIG_NET_SOCKETS_TLS_PRIORITY=35 # Modem library CONFIG_NRF_MODEM_LIB=y CONFIG_NRF_MODEM_LIB_ON_FAULT_APPLICATION_SPECIFIC=y # LTE connectivity with network connection manager CONFIG_LTE_CONNECTIVITY=y CONFIG_NET_CONNECTION_MANAGER=y CONFIG_NET_CONNECTION_MANAGER_MONITOR_STACK_SIZE=1024 # Increased sysworkq size, due to LTE connectivity CONFIG_SYSTEM_WORKQUEUE_STACK_SIZE=2048 # Disable options y-selected by NCS for no good reason CONFIG_MBEDTLS_KEY_EXCHANGE_DHE_PSK_ENABLED=n CONFIG_MBEDTLS_KEY_EXCHANGE_DHE_RSA_ENABLED=n # Generate MCUboot compatible images CONFIG_BOOTLOADER_MCUBOOT=y # HCI low power UART CONFIG_NRF_SW_LPUART=y CONFIG_NRF_SW_LPUART_INT_DRIVEN=y CONFIG_UART_2_ASYNC=y CONFIG_UART_2_INTERRUPT_DRIVEN=n CONFIG_UART_2_NRF_HW_ASYNC=y CONFIG_UART_2_NRF_HW_ASYNC_TIMER=2
Now in the prj.conf
file for the central_ht
code sample, add the following Kconfig symbols. These have the effect of enabling the Golioth SDK, enabling the Stream service, and using runtime settings to store your device credentials in the storage partition using the Zephyr shell. The main stack size is also increased to account for some additional memory usage.
CONFIG_BT=y CONFIG_LOG=y CONFIG_BT_CENTRAL=y CONFIG_BT_SMP=y CONFIG_BT_GATT_CLIENT=y CONFIG_CBPRINTF_FP_SUPPORT=y # Golioth Firmware SDK CONFIG_GOLIOTH_FIRMWARE_SDK=y # Application CONFIG_MAIN_STACK_SIZE=2048 CONFIG_GOLIOTH_SAMPLE_COMMON=y CONFIG_LOG_BACKEND_GOLIOTH=y CONFIG_GOLIOTH_SETTINGS=y CONFIG_GOLIOTH_STREAM=y CONFIG_GOLIOTH_SAMPLE_HARDCODED_CREDENTIALS=n CONFIG_FLASH=y CONFIG_FLASH_MAP=y CONFIG_NVS=y CONFIG_SHELL=y CONFIG_SETTINGS=y CONFIG_SETTINGS_RUNTIME=y CONFIG_GOLIOTH_SAMPLE_PSK_SETTINGS=y CONFIG_GOLIOTH_SAMPLE_SETTINGS_AUTOLOAD=y CONFIG_GOLIOTH_SAMPLE_SETTINGS_SHELL=y CONFIG_LOG=y CONFIG_EVENTFD_MAX=14 CONFIG_LOG_PROCESS_THREAD_STACK_SIZE=1536 CONFIG_MBEDTLS_ENABLE_HEAP=y CONFIG_MBEDTLS_HEAP_SIZE=10240 CONFIG_MBEDTLS_SSL_IN_CONTENT_LEN=2048 CONFIG_MBEDTLS_SSL_OUT_CONTENT_LEN=2048 CONFIG_NETWORKING=y CONFIG_NET_IPV4=y CONFIG_POSIX_MAX_FDS=23
3. Add Golioth API calls to main.c
With all the configuration in place, we’re now ready to update main.c
to connect to Golioth and push temperature readings to the cloud.
First, add some includes, create a semaphore, and add a callback near the top of main.c
:
#include <golioth/client.h> #include <golioth/stream.h> #include <samples/common/net_connect.h> #include <samples/common/sample_credentials.h> static struct golioth_client *client; static K_SEM_DEFINE(golioth_connected, 0, 1); static void on_client_event(struct golioth_client *client, enum golioth_client_event event, void *arg) { bool is_connected = (event == GOLIOTH_CLIENT_EVENT_CONNECTED); if (is_connected) { k_sem_give(&golioth_connected); } printk("Golioth client %s\n", is_connected ? "connected" : "disconnected"); }
Next, in the notify_func()
function, add an API call to send data to Golioth:
char sbuf[32]; snprintk(sbuf, sizeof(sbuf), "{\"temperature\":%g}", temperature); printf("Sending to Golioth: %s\n", sbuf); int err = golioth_stream_set_async(client, "sensor", GOLIOTH_CONTENT_TYPE_JSON, sbuf, strlen(sbuf), NULL, NULL); if (err) { printf("Failed to push temperature: %d\n", err); }
Finally, in main()
add code to start the Golioth client connection:
char sbuf[32]; snprintk(sbuf, sizeof(sbuf), "{\"temperature\":%g}", temperature); printf("Sending to Golioth: %s\n", sbuf); int err = golioth_stream_set_async(client, "sensor", GOLIOTH_CONTENT_TYPE_JSON, sbuf, strlen(sbuf), NULL, NULL); if (err) { printf("Failed to push temperature: %d\n", err); }
Running the demo and viewing data on the cloud
The first time you run the nRF9160-DK you need to add device credentials. Open a serial terminal to the device and use the shell to issue the following commands:
uart:~$ settings set golioth/psk-id my-psk-id@my-project uart:~$ settings set golioth/psk my-psk uart:~$ kernel reboot warm
With both of our boards programmed and powered on, we can view the terminal output of the nRF9160-DK to see the connection and scanning process:
*** Booting nRF Connect SDK v2.5.2 *** [00:00:00.465,972] <inf> fs_nvs: 2 Sectors of 4096 bytes [00:00:00.466,003] <inf> fs_nvs: alloc wra: 0, fb8 [00:00:00.466,003] <inf> fs_nvs: data wra: 0, 68 [00:00:00.466,278] <inf> golioth_samples: Bringing up network interface [00:00:00.466,308] <inf> golioth_samples: Waiting to obtain IP address [00:00:02.545,684] <inf> lte_monitor: Network: Searching [00:00:05.688,049] <inf> lte_monitor: Network: Registered (roaming) [00:00:05.689,117] <inf> golioth_mbox: Mbox created, bufsize: 1232, num_items: 10, item_size: 112 [00:00:07.861,846] <inf> golioth_coap_client_zephyr: Golioth CoAP client connected Golioth client connected [00:00:07.862,365] <inf> golioth_coap_client_zephyr: Entering CoAP I/O loop [00:00:08.559,051] <wrn> bt_hci_core: opcode 0x0000 pool id 5 pool 0x2000d430 != &hci_cmd_pool 0x2000d488 [00:00:08.600,585] <inf> bt_hci_core: HW Platform: Nordic Semiconductor (0x0002) [00:00:08.600,616] <inf> bt_hci_core: HW Variant: nRF52x (0x0002) [00:00:08.600,646] <inf> bt_hci_core: Firmware: Standard Bluetooth controller (0x00) Version 141.732 Build 3324398027 [00:00:08.610,504] <inf> bt_hci_core: Identity: E1:99:6F:78:C4:C4 (random) [00:00:08.610,534] <inf> bt_hci_core: HCI: version 5.4 (0x0d) revision 0x1168, manufacturer 0x0059 [00:00:08.610,565] <inf> bt_hci_core: LMP: version 5.4 (0x0d) subver 0x1168 Bluetooth initialized Scanning successfully started [DEVICE]: F4:BC:DA:35:5E:68 (public), AD evt type 0, AD data len 27, RSSI -85 [AD]: 1 data_len 1 [AD]: 9 data_len 12 [AD]: 255 data_len 8 [DEVICE]: F4:BC:DA:35:5E:68 (public), AD evt type 4, AD data len 31, RSSI -85 [DEVICE]: F0:5F:8B:2D:1C:A2 (random), AD evt type 0, AD data len 20, RSSI -82 [AD]: 9 data_len 5 [AD]: 25 data_len 2 [AD]: 1 data_len 1 [AD]: 2 data_len 4 [DEVICE]: F0:5F:8B:2D:1C:A2 (random), AD evt type 4, AD data len 0, RSSI -81 [DEVICE]: E9:E3:F1:6D:A9:87 (random), AD evt type 0, AD data len 11, RSSI -29 [AD]: 1 data_len 1 [AD]: 3 data_len 6 uart:~$ Temperature 21.5C. Sending to Golioth: {"temperature":21.5} Connected: E9:E3:F1:6D:A9:87 (random) [ATTRIBUTE] handle 25 [ATTRIBUTE] handle 26 [ATTRIBUTE] handle 28 [SUBSCRIBED] uart:~$ Temperature 21.5C. Sending to Golioth: {"temperature":21.5} Temperature 21.25C. Sending to Golioth: {"temperature":21.25} Temperature 21.5C. Sending to Golioth: {"temperature":21.5} Temperature 21.25C. Sending to Golioth: {"temperature":21.25}
We see the chip boot, connect to the cell network, then connect to Golioth. After that, the Bluetooth scan begins, connecting to devices it finds to query for the desired health temperature service (HTS). Once a service is found, we see temperature readings that are then pushed to the cloud.
Checking on the LightDB Stream tab in the device view of the Golioth web console shows the data arriving on the cloud!
Connecting Bluetooth to the Cloud
This lays the groundwork for connecting your Bluetooth devices to the cloud. One gateway (or a relatively small number of them) can service multiple BLE peripheral devices for both read and write activities. It’s even possible to update firmware over a Bluetooth connection. But that’s a post for a different day.
Give Golioth a try, it’s free for individuals! If you have a need for a more involved Bluetooth to Cellular gateway, we’d love to hear about it. Reach out to the Golioth DevRel team.
If you want to dive into the code from this post, start with the Zephyr central_ht sample and apply the file changes found in this gist.